Note
Go to the end to download the full example code
Vertical extrapolation
Example of using PyWAsP to perform vertical extrapolation.
Prepare TopographyMap
First, we need to prepare the topography map. This is done by reading the elevation and roughness maps and creating a TopographyMap object.
import numpy as np
import windkit as wk
import pywasp as pw
bwc = wk.read_bwc(
"../../../modules/examples/tutorial_1/data/SerraSantaLuzia.omwc", crs="EPSG:4326"
)
bwc = wk.spatial.reproject(bwc, to_crs="EPSG:32629")
elev_map = wk.read_vector_map(
"../../../modules/examples/tutorial_1/data/SerraSantaLuzia.map",
map_type="elevation",
crs="EPSG:32629",
)
lc_map, lc_tbl = wk.read_vector_map(
"../../../modules/examples/tutorial_1/data/SerraSantaLuzia.map",
map_type="roughness",
crs="EPSG:32629",
)
topo_map = pw.wasp.TopographyMap(elev_map, lc_map, lc_tbl)
Define output locations
Second, we need to define the output locations. This is done by creating a dataset with the coordinates of the output locations.
output_locs = wk.create_dataset(
west_east=bwc.west_east.values,
south_north=bwc.south_north.values,
height=np.linspace(10.0, 100.0, 10),
crs="EPSG:32629",
struct="stacked_point",
)
print(output_locs)
<xarray.Dataset>
Dimensions: (height: 10, stacked_point: 1)
Coordinates:
* height (height) float64 10.0 20.0 30.0 40.0 ... 70.0 80.0 90.0 100.0
south_north (stacked_point) float64 4.621e+06
west_east (stacked_point) float64 5.147e+05
crs int8 0
Dimensions without coordinates: stacked_point
Data variables:
output (height, stacked_point) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0
Attributes:
Conventions: CF-1.8
history: 2024-06-11T13:35:12+00:00:\twindkit==0.8.0\twk.create_dataset(
Calculate resource grid
Finally, we can calculate the resource grid. This is done by calling the predict_wwc function. This function takes the output locations, the boundary conditions, and the topography map as input. The output is a weibull wind climate dataset at the output locations.
wwc = pw.wasp.predict_wwc(bwc, topo_map, output_locs)
<frozen importlib._bootstrap>:241: RuntimeWarning: numpy.ndarray size changed, may indicate binary incompatibility. Expected 16 from C header, got 96 from PyObject
/opt/conda/lib/python3.11/site-packages/windkit/spatial/_bbox.py:263: UserWarning: 'use_bounds' currently defaults to False in BBox.reproject, in the future this will change to True
warnings.warn(
/opt/conda/lib/python3.11/site-packages/windkit/spatial/_bbox.py:263: UserWarning: 'use_bounds' currently defaults to False in BBox.reproject, in the future this will change to True
warnings.warn(
/opt/conda/lib/python3.11/site-packages/windkit/spatial/_bbox.py:263: UserWarning: 'use_bounds' currently defaults to False in BBox.reproject, in the future this will change to True
warnings.warn(
/opt/conda/lib/python3.11/site-packages/windkit/spatial/_bbox.py:263: UserWarning: 'use_bounds' currently defaults to False in BBox.reproject, in the future this will change to True
warnings.warn(
Plot the mean wind speed
We can plot the mean wind speed to see the result.
wwc["wspd"].plot.line(y="height")
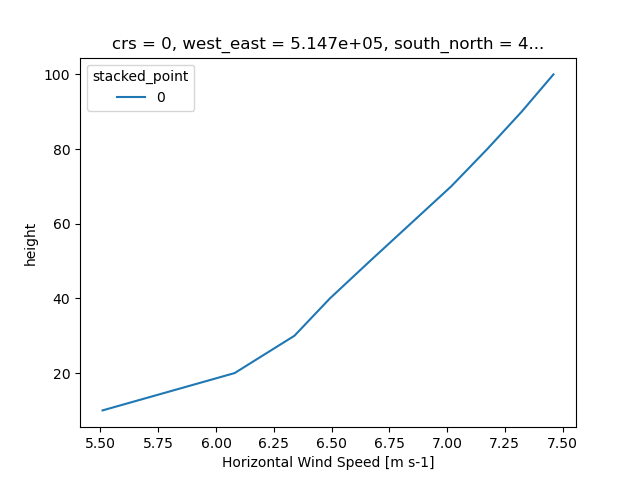
[<matplotlib.lines.Line2D object at 0x7f8538d25490>]
Total running time of the script: (0 minutes 5.071 seconds)